Options
Homework - Stephanie Hedger
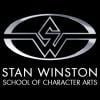
nspiration
I've decided to take inspiration from a book called Frontier Incursion, a Sci-Fi book with a species called Starcats. Which are basically large cats that have glowing marks along their bodies.
Goal
Instead of trying to recreate the entire cat (for now) I've decided to focus solely on their ears. So I've created them out of fabric and cardboard while the skull top is paper mache and will focus on the movement of the ears.
I'm using the mechanism ideas from the plastic bag monsters course in creating the movement within the ears themselves allowing me to create different emotions based on position: relaxed, calm, angry and agitated.
(Sorry the photo's are sideways they're right on the computer I can't find a way to change them here)
Calm

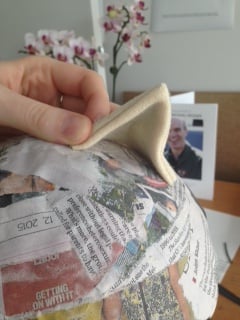
Alert
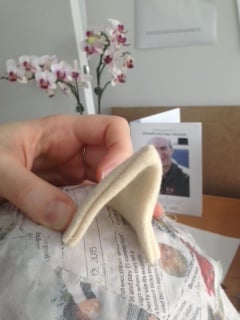
Angry
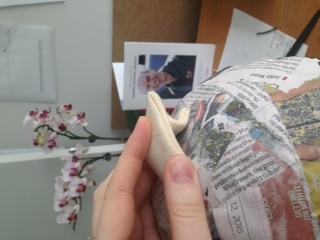
I am planning on using 3 servos for each ear but as they have not arrived yet I have also ordered 2 pan and tilt servos that I may be able to use instead. Which may save space.
They will be placed so one servo controls the 'calm/alert' state while, another controls the 'angry' state and the third should make the tip twitch as if the cat is agitated. (This would obviously be duplicated on each ear.)
I have an Arduino Uno that I am going to use for this version though for a later iteration I may require a smaller board. This will allow me the full range of inputs and outputs available on the board though I should not need them.
Electronic Components
-6 Servos or 2 Servos + 2 Pan & Tilt Servos
Mechanical Components
-Cat's Head
-Cat's ears x2
-Pivot Attachments
-Motivators (Likely cotton if strong enough)
Bonus Goal 1: Making the ears light up.
I plan on using black light paint with UV LEDs to illuminate it
Electronic Components
-UV LEDs
Mechanical Components
-UV Paint
-Fibre Optics (Possibly)
Bonus Goal 2: Sound reaction
I would also like to add a small microphone to the ears so the will automatically twitch based on sound. This will depend on component availability and coding difficulty in the time frame.
Electronic Components
-Microphone or speaker
I've decided to take inspiration from a book called Frontier Incursion, a Sci-Fi book with a species called Starcats. Which are basically large cats that have glowing marks along their bodies.
Goal
Instead of trying to recreate the entire cat (for now) I've decided to focus solely on their ears. So I've created them out of fabric and cardboard while the skull top is paper mache and will focus on the movement of the ears.
I'm using the mechanism ideas from the plastic bag monsters course in creating the movement within the ears themselves allowing me to create different emotions based on position: relaxed, calm, angry and agitated.
(Sorry the photo's are sideways they're right on the computer I can't find a way to change them here)
Calm
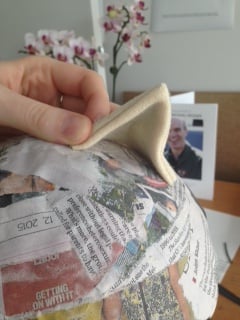
Alert
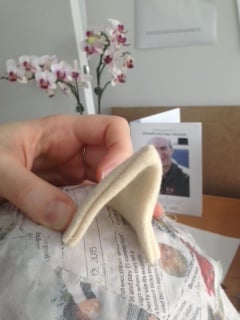
Angry
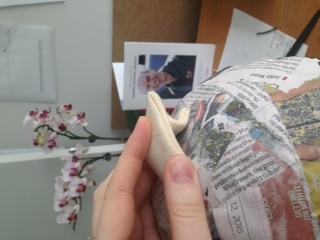
I am planning on using 3 servos for each ear but as they have not arrived yet I have also ordered 2 pan and tilt servos that I may be able to use instead. Which may save space.
They will be placed so one servo controls the 'calm/alert' state while, another controls the 'angry' state and the third should make the tip twitch as if the cat is agitated. (This would obviously be duplicated on each ear.)
I have an Arduino Uno that I am going to use for this version though for a later iteration I may require a smaller board. This will allow me the full range of inputs and outputs available on the board though I should not need them.
Electronic Components
-6 Servos or 2 Servos + 2 Pan & Tilt Servos
Mechanical Components
-Cat's Head
-Cat's ears x2
-Pivot Attachments
-Motivators (Likely cotton if strong enough)
Bonus Goal 1: Making the ears light up.
I plan on using black light paint with UV LEDs to illuminate it
Electronic Components
-UV LEDs
Mechanical Components
-UV Paint
-Fibre Optics (Possibly)
Bonus Goal 2: Sound reaction
I would also like to add a small microphone to the ears so the will automatically twitch based on sound. This will depend on component availability and coding difficulty in the time frame.
Electronic Components
-Microphone or speaker
1
Comments
Unfortunately because it's made from cardboard all the testing has compromised its structural integrity. I think I've found a maker space that I can 3d print a plastic version that should work much better but for the minute here's a paper mache cat.
Here is a video of it working currently.